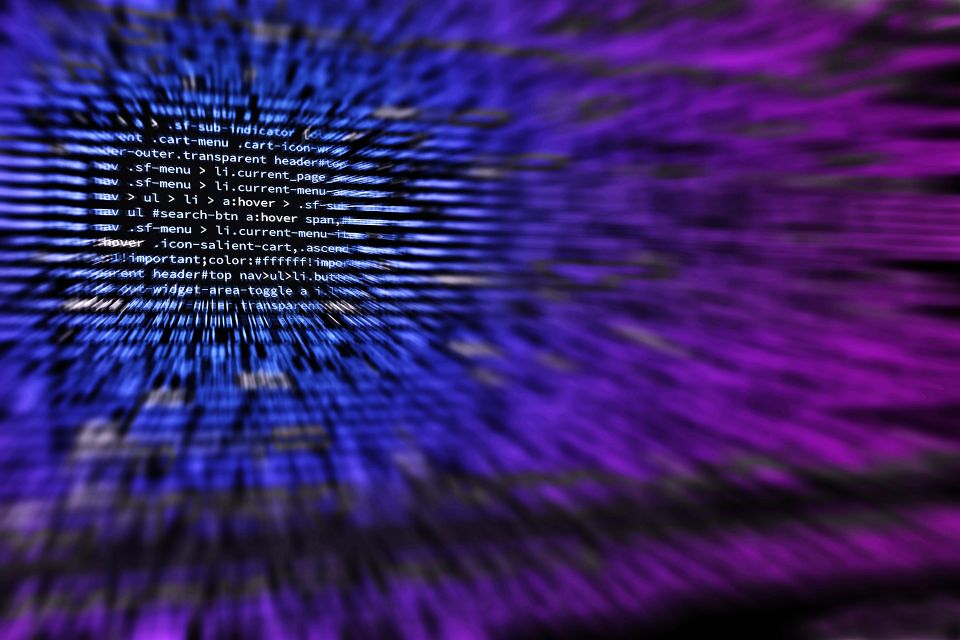
Understanding the Basics of HTML, CSS, and JavaScript
When it comes to web development, three fundamental technologies are essential for creating and maintaining websites: HTML, CSS, and JavaScript. These languages work together to build the structure, style, and interactivity of a web page, making them the foundation of web development. Whether you're a beginner looking to understand how websites work or a business owner wanting to better communicate with your web development team, understanding the basics of HTML, CSS, and JavaScript is crucial. In this blog post, we’ll break down the core concepts of each language and how they contribute to building a website.
1. HTML: The Structure of the WebHTML (HyperText Markup Language) is the backbone of any website. It is used to create the basic structure of a web page by defining its content and layout. HTML uses a series of elements and tags to tell the browser how to display text, images, links, and other media.
Key Concepts in HTML:
- Elements and Tags: HTML consists of elements that are enclosed in tags. Tags are written in angle brackets (e.g., <tag>), and most tags come in pairs with an opening tag and a closing tag (e.g., <p></p> for a paragraph). Elements can include headings, paragraphs, links, images, and more.
- Attributes: Tags can have attributes that provide additional information about an element. For example, the href attribute in an <a> tag defines the URL of the link: <a href="https://example.com">Click Here</a>.
- Nesting: HTML elements can be nested within other elements to create a structured layout. For example, a list (<ul>) can contain multiple list items (<li>).
- HTML5: The latest version of HTML, HTML5, introduces new elements and attributes for better semantics, multimedia handling, and mobile support, such as <header>, <footer>, <video>, and <article>.
html
<!DOCTYPE html> <html> <head> <title>My First Web Page</title> </head> <body> <h1>Welcome to My Website</h1> <p>This is a simple web page created using HTML.</p> <a href="https://example.com">Learn more</a> </body> </html>
In this example, the <html> element wraps the entire content of the page, <head> contains meta-information like the page title, and <body> includes the visible content, such as headings, paragraphs, and links.
2. CSS: Styling the Web
CSS (Cascading Style Sheets) is a language used to control the visual presentation of a web page. While HTML provides the structure, CSS is responsible for the look and feel, including layout, colors, fonts, and spacing. CSS allows developers to create visually appealing websites and ensures a consistent design across all pages.
Key Concepts in CSS:
- Selectors: CSS applies styles to HTML elements using selectors. A selector targets specific elements on the page, such as tags, classes, or IDs. For example, p selects all paragraph elements, while .className selects elements with a specific class.
- Properties and Values: CSS styles are defined by properties and values. Properties specify what aspect of the element to style (e.g., color, font-size, margin), and values define how it should be styled (e.g., color: red;).
- Classes and IDs: Classes and IDs are used to apply styles to specific elements. Classes are reusable across multiple elements and are denoted by a period (e.g., .button). IDs are unique to a single element and are denoted by a hash symbol (e.g., #header).
- Box Model: The CSS box model describes how elements are rendered, including margins, borders, padding, and content. Understanding the box model is essential for controlling layout and spacing on the page.
- Responsive Design: CSS includes features like media queries that allow developers to create responsive designs, which adapt the layout to different screen sizes (e.g., desktop, tablet, mobile).
css
body { font-family: Arial, sans-serif; background-color: #f4f4f4; margin: 0; padding: 20px; } h1 { color: #333; font-size: 24px; } a { color: #007BFF; text-decoration: none; } a:hover { text-decoration: underline; }
In this example, the body selector styles the overall page, setting the font family, background color, margin, and padding. The h1 selector styles the heading, and the a selector styles links, including a hover effect.
3. JavaScript: Interactivity on the Web
JavaScript is a powerful programming language that enables interactivity and dynamic behavior on web pages. While HTML and CSS are used to create static content and design, JavaScript allows developers to create interactive elements, such as forms, animations, and real-time updates.
Key Concepts in JavaScript:
- Variables and Data Types: JavaScript uses variables to store data, which can be of various types, such as strings (text), numbers, booleans (true/false), and objects. Variables are declared using var, let, or const.
- Functions: Functions are blocks of code that perform specific tasks. They can be defined and called to execute actions, such as manipulating the DOM (Document Object Model), handling events, or performing calculations.
- DOM Manipulation: JavaScript interacts with HTML through the DOM, which represents the structure of the web page. Developers can use JavaScript to access and modify HTML elements, such as changing text, styles, or attributes dynamically.
- Events: JavaScript responds to user interactions through events, such as clicks, form submissions, or keyboard inputs. Event listeners are used to execute code when a specific event occurs (e.g., a button click).
- Loops and Conditionals: JavaScript includes control structures like loops (for, while) and conditionals (if, else) to execute code based on conditions or repeatedly perform tasks.
javascript
// Display an alert message when the page loads window.onload = function() { alert('Welcome to My Website!'); }; // Change the text of a paragraph when a button is clicked function changeText() { document.getElementById('myParagraph').innerText = 'You clicked the button!'; }
In this example, the window.onload event triggers an alert message when the page loads. The changeText function changes the text of a paragraph with the ID myParagraph when a button is clicked.
4. How HTML, CSS, and JavaScript Work Together
HTML, CSS, and JavaScript are used in tandem to create fully functional and visually appealing websites. HTML provides the structure, CSS handles the styling, and JavaScript adds interactivity. Here’s how they work together:
- HTML defines the basic structure of the page, such as headings, paragraphs, images, and links.
- CSS styles these elements, determining their appearance, layout, colors, and fonts.
- JavaScript adds interactivity by responding to user actions, updating content dynamically, and enhancing user experience.